What is an HKN-Sigma Resume Book?
As part of our industry relations, HKN is happy to provide a Resume Book of our members. We saw the need for this book, as HKN students could not find a suitable yet personal way to connect with employers without being subjected to hundreds of web requests from unfitting companies. Likewise, we hope companies will find our book a rich resource of high-caliber future employees. As our school motto implies, we certainly believe that "our hearts are in the work."
What are the demographics?
Our Resume Book has over 30 current HKN members, taken from the top 25% of the CMU ECE junior class, the top 33% of the senior ECE class, the top 33% of the masters class, and distinguished ECE doctoral students. Our demographics are as diverse as the ECE community at Carnegie Mellon: undergraduate students, graduate students (accelerated M.S., pure M.S., and Ph.D. ), U.S. Citizens, and international students -- all spanning a considerable number of ECE concentrations and proficiencies.
How often is it updated?
Members are asked if they would like to participate in the book once every two months. Likewise, if a member finds employment or graduates, they are removed from the Resume Book.
How much does the Resume Book cost? How is it delivered?
We ask for a donation of $150 to obtain the most recent resume book revision. This donation supports our activities, such as mentoring and social events, which helps build a thriving ECE community. Companies may also sponsor HKN events, such an information sessions or hack events. These events are great for getting in touch with our society; we will also list sponsoring companies on our website, provide them with a free Resume Book, and proclaim their greatness throughout the Halls of Hamerschlag.
How is it delivered?
The resume book is typically delivered via email, in the form of a *.pdf document. However, we are more than happy to accommodate a variety of other formats and delivery methods.
Great. How do I order the HKN-Sigma Resume Book?
The best way to order a resume book is to send an e-mail to hkn-officers@lists.andrew.cmu.edu. Our officers will then get in contact with you to complete the transaction. Just as our members are held to high standards, our officers make sure only to send high-quality opportunities to our members to avoid spamming them. This e-mail may also be used for inquiries, such as sponsoring a lunch to eat with a few members, wanting to share opportunities within your company, or hoping to provide HKN sweatshirts with your company's logo splashed all over it. We are very happy to talk to companies--please let us know you are out there!
What is an Information Session?
An information session (or, simply, "Infosession" ) is a recruiting event that's sponsored by an interested company. This allows employers to establish a campus presence and collect resumes from some of the most-hardworking ECE students in the field, and it provides Carnegie Mellon ECE students a chance to explore various opportunities in industry. This event typically involves a formal presentation from the company, followed by a less-formal food mixer.
How is it marketed? Is HKN-Sigma willing to co-sponsor?
The event advertisement will be distributed throughout the department via email broadcast, event postings on this website, and physical flyer postings throughout Hamerschlag Hall (ECE department home) on Carnegie Mellon campus. In addition to marketing the infosession, we are willing to assist in any other logistics involved with scheduling and setting the event up. This must be specifically coordinated with our organization, and -- considering various scheduling demands -- we ask for an additional gift accordingly.
What are the venue details and projected turnout?
According to availability of locations, Information Sessions are generally scheduled in Hamerschlag Hall (ECE), Gates & Hillman Center (Computer Science), or Newell Simon Hall (Robotics Institute, Human Computer Interaction, Quality of Life). We will try our best to accommodate any specific room requests you may have. All of these locations are furnished with at least two whiteboards and an LCD projector, but, if additional equipment is needed, we can usually accommodate the request also (given enough notice). In order to maximize the projected student turnout (~50 students), scheduling the event after 4:30pm on Tuesdays and Thursdays is suggested, although Mondays and Wednesdays after 4:30pm are feasible as well. Providing food and even small company souvenirs are customary; we are willing to offer suggestions, to help provide the students with the best, most unique experience possible.
How much does it cost? How do I sign up?
We ask for a tax-dedictible donation of $500 (excluding food, equipment rental expenses, and co-sponsorship expenses), but more generosity is appreciated. We are a 501(c)3 tax-exempt non-profit, university-affiliated organization; we use these funds to improve our services to the ECE community. To schedule an infosession, please email us at hkn-officers@lists.andrew.cmu.edu.
This e-mail may also be used for inquiries, such as sponsoring a lunch to eat with a few members, wanting to share opportunities within your company, or hoping to provide HKN sweatshirts with your company's logo splashed all over it. We are very happy to talk to companies -- please let us know you are out there!
What does it mean to "Sponsor" HKN-Sigma?
Sponsoring HKN-Sigma is a unique way for a company to build strong connections with the ECE community. By funding social events and HKN swag, a company can become part of building a bigger and better engineering community. Think back to your college days, and how much fun it was to meet friends outside the lab, and build friendships that last long beyond graduation.
One of HKN's goals is to foster a community, and we feel industry should certainly be part of this community. Companies wishing to fund activities such as snack-night, study breaks, dinners, tech talks, meet & mingles, half-off nights in Oakland (support can go towards currently existing activities or new, company-specific events!) or swag (t-shirts, hoodies, polos, etc.) can contact us personally. Welcome to the community!
How does HKN-Sigma use sponsorship funds?
Sponsorship funds go directly towards food, swag, and location bookings. A company may be specific in how its funds should be dispersed, or can simply donate in good measure. Typically, a full semester's support of snack night is ~$1500 for fifteen weeks. Snack night is held once a week, during labs. We also hope to further support community-building events, such as study breaks, info sessions, and meet & mingles. An example would be a quick study break for any HKN members to go to Razzy Fresh, a local frozen-yogurt shop, or Chipotle, for their famed burritos. These events are currently paid for by the members themselves, but we would be ecstatic for industry members to join us. We also are always open for a company to sponsor HKN hoodies, which are quite popular. The company's logo will be prominent on the T-shirt. Sponsorship funds are not given to students directly, but rather dispersed through the ECE Department's Alumni Relations Office. This ensures accountability and diligence in funding events.
Why would I want to sponsor HKN-Sigma?
As the premiere Electrical & Computer Engineering Honor Society, our members have proven to be some of the best engineers Carnegie Mellon has to offer. Our membership is also extremely diverse in their interests: We have members in all fields of ECE, including computer architecture, embedded systems, applied physics / semiconductors, signal processing / controls, and software. One of HKN's goal is to bring such diversity together, so that we may learn from each other in both casual and academic settings. We also treasure company experiences, as being exposed to industry is a strong component of the CMU education. By sponsoring HKN events, you further enable this internal department communication and collaboration, via our company-sponsored snack night events -- where more than 50 ECE students (including undergraduate, masters, and PhD students) can enjoy each other's company over a beverages and snacks. While it may be a simple gesture, snack night has become part of ECE culture, with students planning their schedules around it. Thus, we greatly welcome companies to join in as well.
Cool. How do I become an HKN-Sigma sponsor?
Please contact our current officers at hkn-officers@lists.andrew.cmu.edu to discuss what you would like to sponsor. We will then get you in touch with our ECE Department contact, which can set up the terms of the funding and any financial transactions.
For sponsoring our events, we acknowledge the company's presence at each event, on our website, and through ensignia on the chapter's regalia. We are also thrilled when representatives come to any of our events. We have also noticed that a correlation exists amongst high attendance at infosession and recruiting booths, student enthusiasm due to great food and souveniers, and longterm research partnerships. We greatly appreciate any support, and hope you will be coming to visit us soon!
Sponsor(s), past and present:
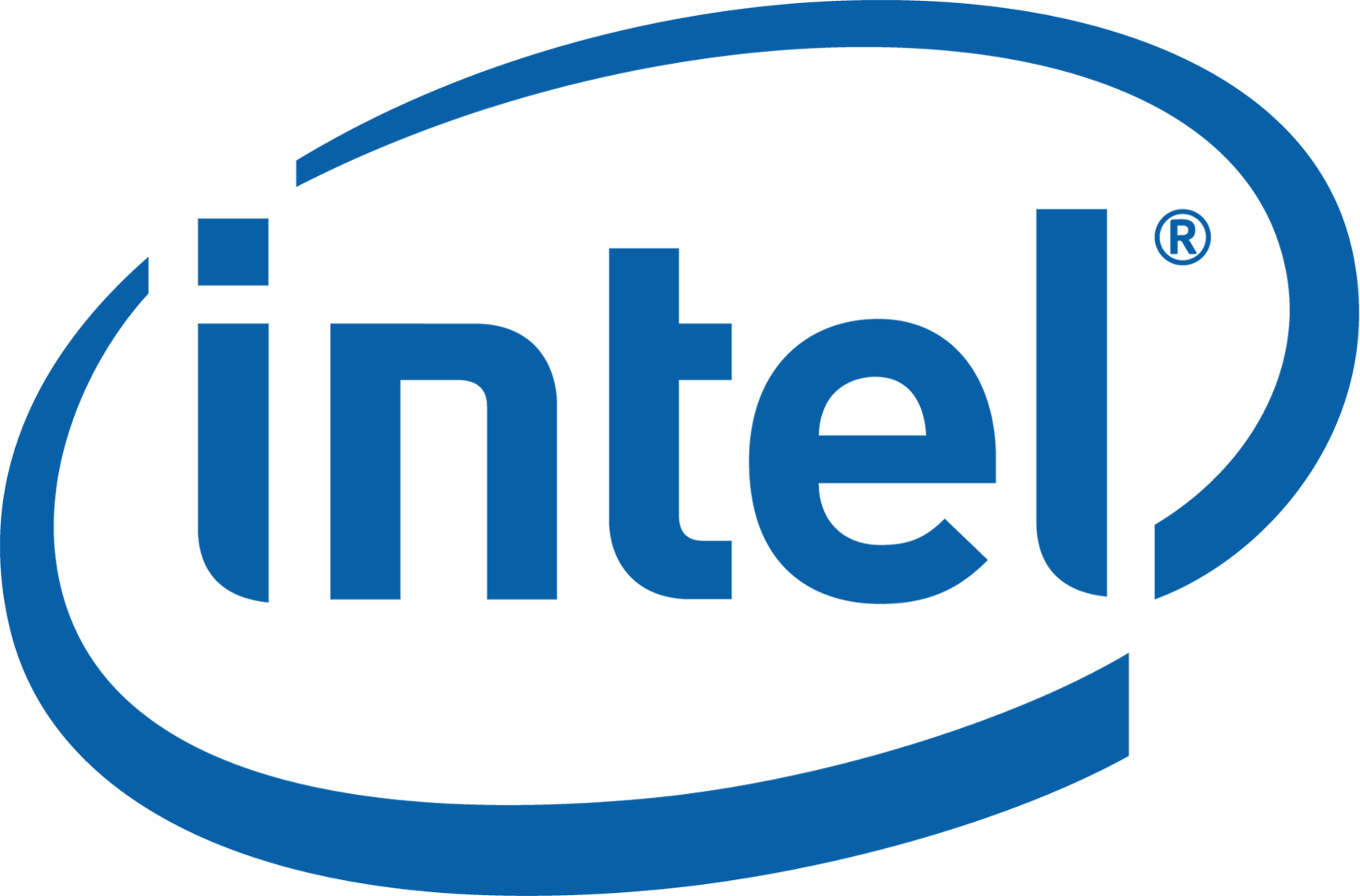

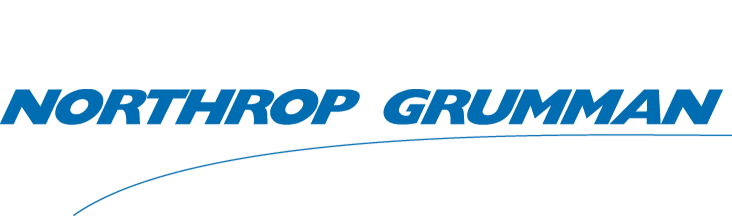
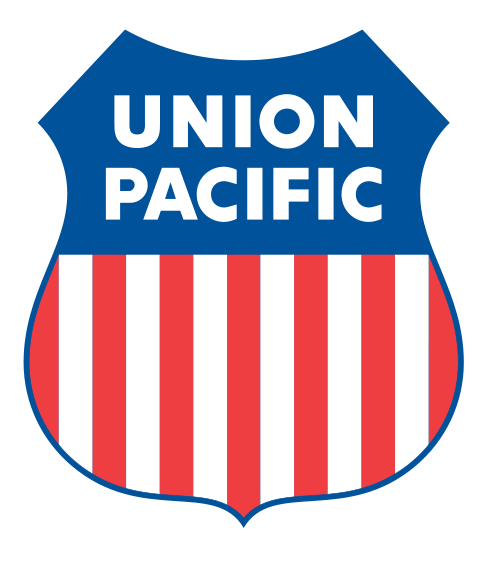
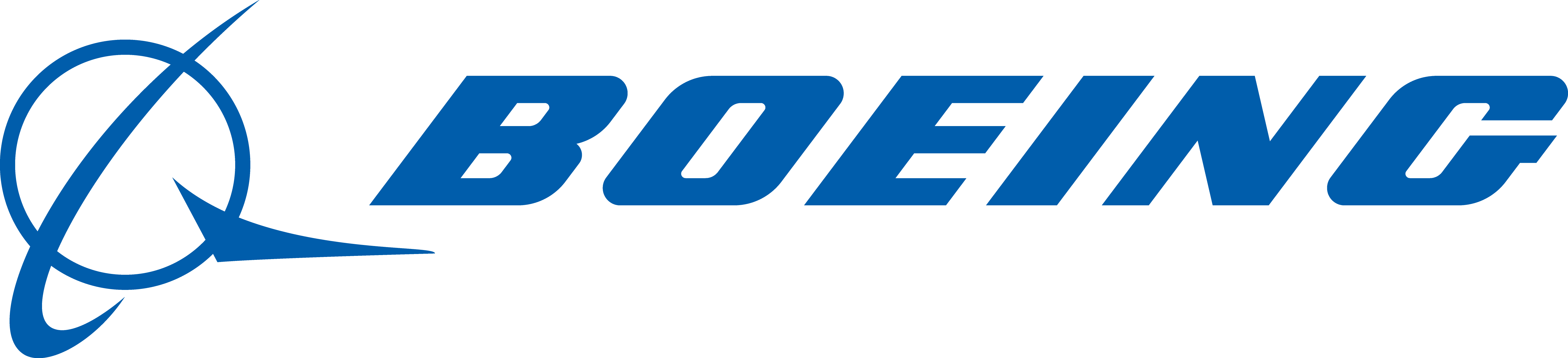

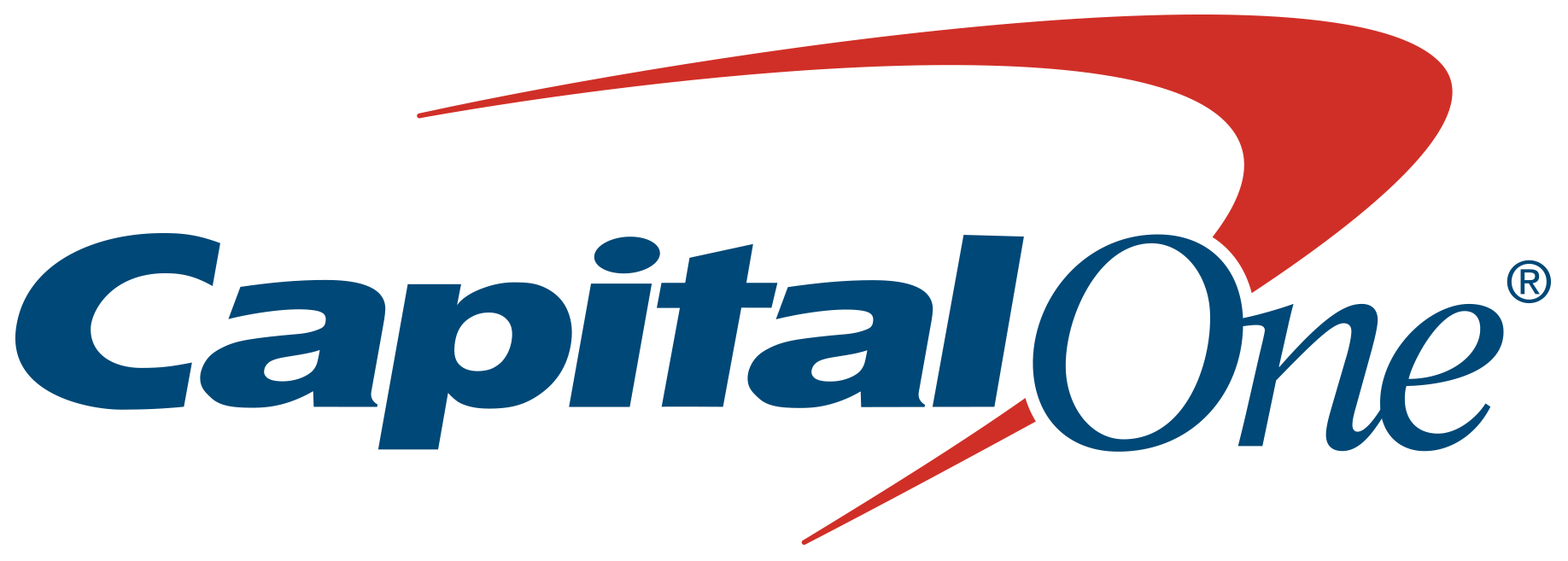